[React] CRA 사용 없이 React/Typescript 개발 환경 구축(with. Webpack/babel)
해당 글에서는 CRA(Create-React-App)를 사용하지 않고 개발 환경을 구축하는 방법에 대해서 공유합니다. 더불어서 선택적으로 설치해야 할 사항까지 함께 공유합니다.
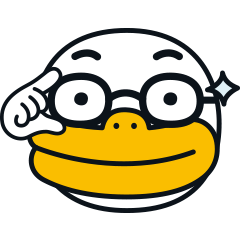
1) 개발 환경
💡 개발 환경 구축을 위해 이용한 IDE, Package Manager, Package를 정리 한 내용 입니다.
분류 | 버전 | 설명 |
Visiual Studio | latest | 개발 IDE 툴로 VSCode를 사용하였습니다. |
node | 16.16.0 | 자바스크립트를 수행하는 용도로 사용하였습니다. |
yarn | 1.22.19 | 패키지 매니저로 사용하였습니다. |
react / react-dom | 18.2.0 | 웹 프레임워크 React와 React-dom을 사용하였습니다. |
typescript | 4.8.4 | 타입스크립트를 사용하였습니다. |
webpack | 5.74.0 | 모듈 번들러로 webpack을 사용하였습니다. |
@babel/core | 7.19.6 | 트랜스 파일러로 babel을 사용하였습니다. |
2) 사전 설치
💡 MacOS의 'Homebrew'를 통하여서 시스템에 설치를 진행 합니다.
# Node Stable Version install
$ brew install node@16
# yarn Pacakage Manager install
$ brew install yarn --ignore-dependencies
# Typescript install
$ brew install typescript@4.8.4
# webpack install
$ brew install webpack
# ESLint install
$ brew install eslint
3) 개발 환경 구성
1. 패키지 매니저 및 패키지 구성
💡 사전에 설치한 패키지 매니저로 'pacakage.json' 파일을 구성한 뒤 주요 패키지들을 설치합니다.
💡 간략한 구성을 위해 Terminal을 이용하여서 디렉터리와 파일들을 생성하도록 작성하였습니다.
# pacakage.json 파일 구성
$ yarn init
# react/react-dom 설치
$ yarn add react react-dom
# index.html 구성
$ mkdir public && public/ && touch index.html
# index.tsx 파일 구성
$ touch index.tsx
# src 폴더 및 App.tsx 파일 구성
$ mkdir src && cd src/ && touch App.tsx && cd ..
[참고] 패키지 매니저 이해하기 - 이전에 작성한 글을 참고하였습니다.
[Node] 자바스크립트 패키지 매니져(npm/yarn) 이해하기 -1
해당 글에서는 자바스크립트의 패키지 매니져를 이해하고 이와 관련된 용어들을 이해하는 글 입니다. 1) 자바스크립트 패키지 매니저(Javascript Package Manager)란? 💡 자바스크립트의 패키지 매니저
adjh54.tistory.com
💡 index.html 파일 구성
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="utf-8" />
<meta name="theme-color" content="#000000" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta http-equiv="cache-control" content="max-age=31536000, no-cache" />
<title>React App</title>
</head>
<body>
<div id="root"></div>
</body>
</html>
[참고] 캐시 무효화를 위해 'cache-control'을 사용하였습니다. - 이전에 작성한 글을 참고하였습니다.
[React] 캐시 무효화(Cache Busting)
해당 글의 목적은 캐시 무효화란 무엇이고 어떠한 해결 방법이 있는지에 알아보기 위한 목적으로 글을 작성하였습니다. 1) 문제점 발생 💡 웹 브라우저에서 새로 배포를 하였는데도 이전 파일
adjh54.tistory.com
💡 index.tsx 파일 구성
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./src/App";
const root = ReactDOM.createRoot(
document.getElementById("root") as HTMLElement
);
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
💡 App.tsx 파일 구성
import React from "react";
const App = () => {
return (
<>
<div>Init Page</div>
</>
);
};
export default App;
💡 1차 디렉터리 구조 구성 결과
2. Typescript 구성하기
💡 Javascript를 정적 타입 형태로 사용하기 위한 목적으로 Typescript로 구성하였습니다.
# 개발 단계로 Typescript를 설치합니다.
yarn add typescript @types/react @types/react-dom --dev
# Typescript 초기화 & tsconfig.json 파일이 구성됩니다.
$ tsc --init
[참고] Typescript 이해하기 - 이전에 작성한 글을 참고하였습니다.
[TS] 타입스크립트(Typescript) 이해하기-1 (정의, 동작원리, 특징)
해당 글은 타입스크립트(Typescript)란 무엇이며, 어떻게 동작이 되고 어떠한 특징을 가지고 있는지에 대해 이해하기 위한 글입니다. 1) 타입스크립트(Typescript) 란? 💡 Microsoft에서 개발하고 유지/관
adjh54.tistory.com
💡 tsconfig.json 파일 구성
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"lib": ["dom", "ES2015", "ES2016", "ES2017", "ES2018", "ES2019", "ES2020"],
"allowJs": true,
"jsx": "react-jsx",
"sourceMap": true,
"outDir": "./dist",
"isolatedModules": true,
"strict": true,
"moduleResolution": "node",
"baseUrl": "./",
"paths": {
"@components/*": ["components/*"],
"@pages/*": ["pages/*"]
},
"allowSyntheticDefaultImports": true,
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true
}
}
💡 [ 꿀팁 ] zsh: command not found: tsc 에러 발생 시 아래 명령어를 입력합니다.
# Typescript를 설치합니다.
$ brew install typescript
# Typescript 버전을 확인합니다.
$ tsc -version
💡 2차 디렉터리 구조 구성 결과
3. Babel 구성하기
# babel 설치
$ yarn add babel-loader @babel/core @babel/preset-env --dev
# Typescript babel 설치
$ yarn add @babel/preset-react @babel/preset-typescript
# babel.config.js 파일 생성
$ touch babel.config.js
💡 babel.config.ts 파일을 구성합니다.
module.exports = {
presets: [
"@babel/preset-react",
"@babel/preset-env",
"@babel/preset-typescript",
],
};
💡 3차 디렉터리 구조 구성 결과
4. webpack 구성하기
# webpack 필수 구성을 설치합니다.
$ yarn add webpack webpack-cli webpack-dev-server webpack-merge --dev
# webpack의 플러그인 관련 구성을 설치합니다
$ yarn add html-webpack-plugin clean-webpack-plugin ts-loader --dev
# webpack 공통 파일을 생성합니다.
$ touch webpack.common.js
💡 webpack.common.js 파일을 구성합니다.
const HtmlWebpackPlugin = require("html-webpack-plugin");
const { CleanWebpackPlugin } = require("clean-webpack-plugin");
const path = require("path");
module.exports = {
entry: "./index.tsx",
resolve: {
extensions: [".js", ".jsx", ".ts", ".tsx"],
},
module: {
rules: [
{
test: /\\.tsx?$/,
use: ["babel-loader", "ts-loader"],
},
{
test: /\\.(png|jpe?g|gif)$/,
use: [
{
loader: "file-loader",
},
],
},
],
},
output: {
path: path.join(__dirname, "/dist"),
filename: "bundle.js",
},
plugins: [
new HtmlWebpackPlugin({
template: "./public/index.html",
}),
new CleanWebpackPlugin(),
],
};
💡 webpack.dev.js 파일을 구성합니다.
const { merge } = require("webpack-merge");
const common = require("./webpack.common.js");
module.exports = merge(common, {
mode: "development",
devtool: "eval",
devServer: {
historyApiFallback: true,
port: 3000,
hot: true,
},
});
💡 webpack.prod.js 파일을 구성합니다.
const { merge } = require("webpack-merge");
const common = require("./webpack.common.js");
module.exports = merge(common, {
mode: "production",
devtool: "hidden-source-map",
});
[참고] Webpack 이해하기 - 이전에 작성한 글을 참고하였습니다.
[JS/Library] Webpack 이해하기 - 1 : 주요 용어
해당 글에서는 Module 번들과 Webpack에 대해 이해하고 webpack에 주요 옵션에 대해서 이해를 목적으로 작성한 글입니다. 1) 취지 💡 이전 React 프로젝트를 초기에 구성하고자 할 때 CRA(Create-React-App)를
adjh54.tistory.com
💡 4차 디렉터리 구조 구성 결과
5. package.json
"scripts": {
"dev": "webpack-dev-server --config webpack.dev.js --open --hot",
"build": "webpack --config webpack.prod.js",
"start": "webpack --config webpack.dev.js"
},
6. 구성한 프로젝트를 실행합니다.
# 구성한 프로젝트를 수행합니다.
$ yarn dev
4) [선택] 기타 설치 사항
1. gitignore 구성
⭕ IDE 툴이나 각각 언어 및 프레임워크에서 올리지 않아도 될 내용을 제외하는 파일을 구성합니다.
# .gitignore 파일 구성
$ touch .gitignore
# [꿀팁] .gitignore가 적용이 안될때
$ git rm -r --cache .
$ git add .
$ git commit -m "cache"
$ git push
[참고] gitignore 파일 만들기
gitignore.io
Create useful .gitignore files for your project
www.toptal.com
[참고] 상세 구성 방법 - 이전에 작성한 글을 참고하였습니다.
[Github] .gitignore 이해 및 구성 방법
해당 글의 목적은 Repository에서 소스 작업 이후 Commit & Push를 하는 경우에 특정 ‘파일’ 및 ‘경로’를 제외하여 해당 작업을 진행하고자 할때, gitignore 파일을 사용합니다.이에 대해 .gitignore에
adjh54.tistory.com
2. prettier 구성
# prettier install
$ yarn add --dev --exact prettier
# prettier config file 생성
$ touch .prettierrc.js
# prettier 모든 파일 적용
$ yarn prettier --write .
[참고] 상세 구성 방법 - 이전에 작성한 글을 참고하였습니다.
[JS/library] Prettier 환경설정 방법
해당 글은 자바스크립트 라이브러리 'Prettier'에 대해서 이해하고 환경설정을 하기 위한 목적으로 작성된 글입니다. 1) Prettier 이해하기 💡 Prettier는 코드를 읽어 들여서 사용자가 지정한 '옵션'에
adjh54.tistory.com
3. ESLint 구성
# ESLint 환경 구성
$ eslint --init
# ESLint와 Prettier를 함께 사용하기
$ yarn add eslint-config-prettier --dev
# ESLint와 React-hooks를 함께 사용하기
y arn add eslint-plugin-react-hooks --dev
module.exports = {
env: {
browser: true,
es2021: true,
},
extends: [
'plugin:react/recommended',
'eslint:recommended',
'plugin:react/recommended',
'prettier',
],
overrides: [],
parserOptions: {
ecmaVersion: 'latest',
sourceType: 'module',
},
plugins: ['react', 'prettier'],
rules: {},
};
4. .env 파일 구성
💡 CRA를 사용하는 경우. env 파일을 바로 사용할 수 있도록 구성을 해주는 기능이 있었지만 직접 구성을 해야 합니다.
# env 파일 생성
$ touch .env
# typescript dotenv install
$ yarn add @types/dotenv --dev
💡 webpack.config.ts 내에 구성합니다.
const Dotenv = require('dotenv-webpack');
module.exports = merge(common, {
...,
plugins: [new Dotenv()],
});
[참고] Npm Module
dotenv-webpack
A simple webpack plugin to support dotenv.. Latest version: 8.0.1, last published: 3 months ago. Start using dotenv-webpack in your project by running `npm i dotenv-webpack`. There are 452 other projects in the npm registry using dotenv-webpack.
www.npmjs.com
오늘도 감사합니다. 😀