반응형
해당 글에서는 Java에서 객체를 직렬화하여 JSON 문자열로 바꾸거나 JSON 문자열을 역직렬화하여 객체로 변환하는 방법에 대해 알아봅니다.
![]()
1) 라이브러리 설치
💡 라이브러리 설치
- Jackson 라이브러리의 databind 모듈을 기반으로 직렬화, 역직렬화를 수행합니다.
dependencies {
implementation 'com.fasterxml.jackson.core:jackson-databind:2.16.1'
}
https://mvnrepository.com/artifact/com.fasterxml.jackson.core/jackson-databind
2) 직렬화 방법 : Object to JSON String
1. 객체
package com.multiflex.multiflexchatgpt.dto;
import lombok.AccessLevel;
import lombok.Builder;
import lombok.Getter;
import lombok.NoArgsConstructor;
@Getter
@NoArgsConstructor(access = AccessLevel.PROTECTED)
public class PersonDto {
private String id;
private String pw;
private String addr;
@Builder
public PersonDto(String id, String pw, String addr) {
this.id = id;
this.pw = pw;
this.addr = addr;
}
}
2. 직렬화 수행
💡 직렬화 수행
1. 객체의 데이터를 Builder로 구성하였습니다.
2. ObjectMapper 인스턴스화를 합니다.
3. writeValueAsString() 메서드로 직렬화를 수행합니다.
4. 직렬화된 데이터를 출력합니다.
@GetMapping("/3")
public ResponseEntity<Object> serializationApi() {
String result = "";
// [STEP1] 객체의 데이터를 builder로 구성하였습니다.
PersonDto personDto = PersonDto.builder()
.id("exampleId")
.pw("examplePw")
.addr("exampleAddr")
.build();
// [STEP2] ObjectMapper 인스턴스화
ObjectMapper objectMapper = new ObjectMapper();
try {
// [STEP3] 직렬화를 수행합니다.
result = objectMapper.writeValueAsString(personDto);
// [STEP4] 직렬화된 데이터를 출력합니다.
// 직렬화 :: {"id": "exampleId", "pw" : "examplePw", "addr" :"exampleAddr"}
log.debug("직렬화 :: " + result);
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
return new ResponseEntity<>(result, HttpStatus.OK);
}
3. 결과 확인

3) 역 직렬화 방법 : JSON String to Object
1. 객체
💡 객체
- 문자열로 직렬화 된 데이터를 객체 형태로 역 직렬화를 수행합니다.
package com.multiflex.multiflexchatgpt.dto;
import lombok.AccessLevel;
import lombok.Builder;
import lombok.Getter;
import lombok.NoArgsConstructor;
@Getter
@NoArgsConstructor(access = AccessLevel.PROTECTED)
public class PersonDto {
private String id;
private String pw;
private String addr;
@Builder
public PersonDto(String id, String pw, String addr) {
this.id = id;
this.pw = pw;
this.addr = addr;
}
}
2. 역 직렬화 수행
💡 역 직렬화 수행
1. ObjectMapper 인스턴스화를 합니다.
2. 역 직렬화를 수행합니다. : PersonDto 객체와 바인딩하여 동일한 형태로 역직렬화를 수행합니다.
3. 역 직렬화 된 데이터를 출력합니다.
@GetMapping("/4")
public ResponseEntity<Object> serializationApi() {
String result = "";
PersonDto personDto;
try {
// [STEP1] ObjectMapper 인스턴스화를 합니다.
ObjectMapper objectMapper = new ObjectMapper();
// [STEP2] 역 직렬화를 수행합니다. : PersonDto 객체와 바인딩하여 동일한 형태로 역직렬화를 수행합니다.
personDto = objectMapper.readValue(result, PersonDto.class);
// [STEP3] 역 직렬화를 출력합니다.
log.debug("역 직렬화 ::" + personDto);
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
return new ResponseEntity<>(personDto, HttpStatus.OK);
}
3. 결과확인
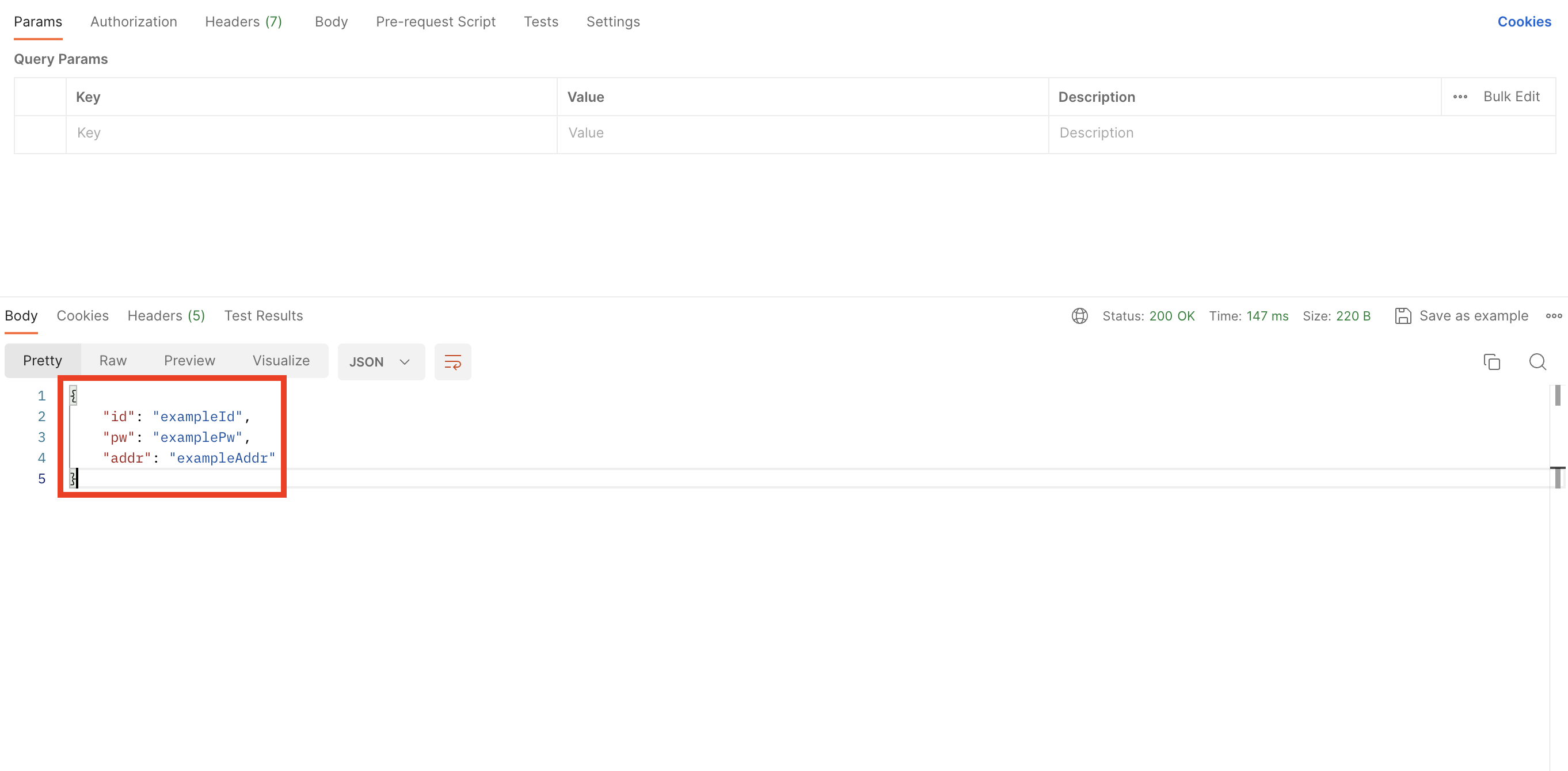
4) Jackson-databind 클래스 및 메서드
클래스 | 메서드 | 설명 |
ObjectMapper | writeValueAsString(value: Any) | 지정된 객체를 JSON 문자열로 직렬화합니다. |
ObjectMapper | readValue(content: String, valueType: TypeReference<T>) | 주어진 JSON 문자열을 지정된 타입의 객체로 변환합니다. |
ObjectMapper | configure() | ObjectMapper의 구성을 설정합니다. |
ObjectMapper | registerModule(module: Module) | 지정된 모듈을 ObjectMapper에 등록합니다. |
ObjectMapper | disable(feature: JsonParser.Feature) | 지정된 JSON 파서 기능을 비활성화합니다. |
ObjectMapper | enable(feature: JsonParser.Feature) | 지정된 JSON 파서 기능을 활성화합니다. |
ObjectMapper | findAndRegisterModules() | 클래스 경로에서 사용 가능한 모듈을 찾아 ObjectMapper에 등록합니다. |
ObjectMapper | writerWithDefaultPrettyPrinter() | 기본 PrettyPrinter를 사용하는 ObjectWriter를 반환합니다. |
ObjectMapper | setVisibilityChecker(checker: VisibilityChecker<*>) | 지정된 가시성 검사기를 ObjectMapper에 설정합니다. |
ObjectMapper | setDefaultPropertyInclusion(inclusion: JsonInclude.Include) | 기본 속성 포함 규칙을 설정합니다. |
ObjectMapper | setSerializationInclusion(inclusion: JsonInclude.Include) | 직렬화 중에 속성을 포함할지 여부를 설정합니다. |
ObjectMapper | setDeserializationInclusion(inclusion: JsonInclude.Include) | 역직렬화 중에 속성을 포함할지 여부를 설정합니다. |
ObjectMapper | configure(feature: SerializationFeature, state: Boolean) | 지정된 직렬화 기능을 설정하거나 해제합니다. |
ObjectMapper | configure(feature: DeserializationFeature, state: Boolean) | 지정된 역직렬화 기능을 설정하거나 해제합니다. |
ObjectMapper | configure(feature: MapperFeature, state: Boolean) | 지정된 매퍼 기능을 설정하거나 해제합니다. |
ObjectMapper | configure(feature: JsonParser.Feature, state: Boolean) | 지정된 JSON 파서 기능을 설정하거나 해제합니다. |
ObjectMapper | configure(feature: JsonGenerator.Feature, state: Boolean) | 지정된 JSON 생성기 기능을 설정하거나 해제합니다. |
ObjectMapper | enableDefaultTyping() | 기본 타입 정보 포함을 활성화합니다. |
ObjectMapper | disableDefaultTyping() | 기본 타입 정보 포함을 비활성화합니다. |
jackson-databind 2.16.1 javadoc (com.fasterxml.jackson.core)
Latest version of com.fasterxml.jackson.core:jackson-databind https://javadoc.io/doc/com.fasterxml.jackson.core/jackson-databind Current version 2.16.1 https://javadoc.io/doc/com.fasterxml.jackson.core/jackson-databind/2.16.1 package-list path (used for ja
www.javadoc.io
오늘도 감사합니다. 😀
반응형
'Java > Short 개발' 카테고리의 다른 글
[Java/Short] IPv4, IPv6 정규 표현식(RegExp) 구성 방법 (4) | 2024.01.29 |
---|---|
[Java/Short] SecureRandom을 이용한 랜덤 숫자/문자(난수) 생성 방법 (0) | 2024.01.22 |
[Java/Short] 문자열의 문자를 가장 앞으로/뒤로 이동하는 방법 (0) | 2023.11.04 |
[Java/Short] 배열을 반으로 나누어 재구성 방법 : for, Arrays.copyOfRange (0) | 2023.11.03 |
[Java/Short] 문자열의 접두사(prefix) / 접미사(suffix) 찾는 방법 : startsWith, endsWith (0) | 2023.09.29 |