해당 글에서는 React Native 환경에서 Drawer Navigation 이해하고 활용하는 방법에 대해 알아봅니다
![]()
1) Drawer Navigation
💡Drawer Navigation
- React Native 앱에서 슬라이드 메뉴(사이드바)를 구현하기 위한 내비게이션 컴포넌트입니다.
- 주로 앱의 메인 메뉴나 섹션 간 이동을 위해 사용됩니다.
기능 | 설명 |
슬라이드 메뉴 | 화면 왼쪽 또는 오른쪽에서 스와이프하여 메뉴를 열 수 있습니다. |
커스터마이징 | 메뉴의 스타일, 애니메이션, 동작 등을 사용자 정의할 수 있습니다. |
제스처 지원 | 스와이프 제스처를 통한 자연스러운 상호작용이 가능합니다. |
중첩 네비게이션 | Stack, Tab 등 다른 네비게이션 타입과 함께 사용할 수 있습니다. |
Drawer Navigator | React Navigation
Drawer Navigator renders a navigation drawer on the side of the screen which can be opened and closed via gestures.
reactnavigation.org
2) Drawer Navigation 활용예시
1. 라이브러리 설치
💡 라이브러리 설치
- Drawer Navigation을 이용하기 위해서는 주요한 라이브러리를 설치합니다.
$ npm install @react-navigation/native @react-navigation/drawer
# or
$ yarn add @react-navigation/native @react-navigation/drawer
$ npm install react-native-gesture-handler react-native-reanimated
# or
$ yarn add react-native-gesture-handler react-native-reanimated
2. App.tsx
💡 App.tsx
- 최초 페이지에서 ApplicationNavigator 컴포넌트를 불러오도록 구성하였습니다.
import React, { useEffect } from "react";
import ApplicationNavigator from "./navigation/ApplicationNavigator";
/**
* Init App
*/
const App = () => {
return (
// {/* Application Navigator */}
<ApplicationNavigator />
);
};
export default App;
3. ApplicationNavigator
💡 ApplicationNavigator
- 앱의 전체 내비게이션 구조를 설정하는 핵심 컴포넌트입니다.
- 아래의 코드에서는 DrawerNavigator를 메인 내비게이터로 사용하고 있으며, 필요에 따라 StackNavigator나 BottomTabNavigator로 쉽게 전환할 수 있도록 구성되어 있습니다.
- NavigationContainer로 전체 내비게이션을 감싸서 내비게이션 상태 관리를 합니다.
- SafeAreaProvider를 통해 iOS의 노치나 홈 인디케이터 등 안전 영역 고려합니다.
- 여러 종류의 네비게이터(Drawer, Stack, BottomTab) 중 선택적으로 사용 가능합니다.
import { NavigationContainer } from "@react-navigation/native";
import React from "react";
import { SafeAreaProvider } from "react-native-safe-area-context";
import DrawerNavigator from "./DrawerNavigator";
import StackNavigator from "./StackNavigator";
import BottomTabNavigator from "./BottomTabNavigator";
const ApplicationNavigator = () => {
return (
<SafeAreaProvider>
<NavigationContainer>
{/* Drawer Navigator를 사용하는 경우 */}
<DrawerNavigator />
{/* Stack Navigator를 사용하는 경우 */}
{/* <StackNavigator /> */}
{/* BottomTab Navigator를 사용하는 경우 */}
{/* <BottomTabNavigator /> */}
</NavigationContainer>
</SafeAreaProvider>
);
};
export default ApplicationNavigator;
4. DrawerNavigator
💡 DrawerNavigator
- createDrawerNavigator()를 사용하여 Drawer 내비게이터를 생성합니다.
💡 기본 설정 : Drawer.Navigator 태그
- initialRouteName: 시작 화면을 HOME으로 지정
- backBehavior: "history" 설정으로 뒤로 가기 동작 정의
- drawerPosition: "left"로 설정하여 왼쪽에서 드로어가 열리도록 구성
💡 주요 화면들 : Drawer.Screen 태그
- 홈, 지난 당첨번호, 당첨번호 분석, 연속 행운번호 생성기, 나의 행운번호 리포트, 알람 설정 순으로 출력이 되도록 구성하였습니다.
import Home from "@/screens/Home";
import WinningHistoryScreens from "@/screens/WinningHistoryScreens";
import { createDrawerNavigator } from "@react-navigation/drawer";
import { Paths } from "./conf/Paths";
import DrawerContentScreen from "./screens/DrawerContentScreen";
import { Text, TouchableOpacity } from "react-native";
import MostWinningNumbersScreen from "@/screens/MostWinningNumbersScreen";
import SaveNumberHisScreen from "@/screens/SaveNumberHisScreen";
import NormalLottoScreen from "@/screens/NormalLottoScreen";
import MultiDrawComponent from "@/screens/MultiDrawComponent";
import LottoNotificationScreen from "@/screens/NotificationLottoScreen";
const Drawer = createDrawerNavigator();
/**
* Drawer Navigator
* @returns
*/
const DrawerNavigator = () => {
// 새로고침 버튼 컴포넌트
const RefreshButton = ({ navigation, routeName }) => {
return (
<TouchableOpacity
onPress={() => {
navigation.reset({
index: 0,
routes: [{ name: routeName }],
});
}}
style={{ marginRight: 15 }}
>
<Text>새로고침</Text>
</TouchableOpacity>
);
};
return (
<Drawer.Navigator
initialRouteName={Paths.HOME}
backBehavior="history"
screenOptions={{
drawerPosition: "left"
}}
>
{/* 메인 화면 */}
<Drawer.Screen
name={Paths.HOME}
component={Home}
options={({ navigation }) => ({
title: "홈",
drawerLabel: "홈",
headerRight: () => (
<RefreshButton navigation={navigation} routeName={Paths.HOME} />
),
})}
/>
{/* 당첨 번호 확인 */}
<Drawer.Screen
name={Paths.LOTTO_WINNING_HIS}
component={WinningHistoryScreens}
options={({ navigation }) => ({
title: "지난 당첨번호",
drawerLabel: "지난 당첨번호",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_WINNING_HIS}
/>
),
})}
/>
<Drawer.Screen
name={Paths.MOST_WINNING_HIS}
component={MostWinningNumbersScreen}
options={({ navigation }) => ({
title: "지난 당첨번호 분석",
drawerLabel: "지난 당첨번호 분석",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.MOST_WINNING_HIS}
/>
),
})}
/>
{/* 기본 번호 생성기 */}
<Drawer.Screen
name={Paths.LOTTO_NORMAL}
component={NormalLottoScreen}
options={({ navigation }) => ({
title: "행운번호 생성기",
drawerLabel: "행운번호 생성기",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_NORMAL}
/>
),
})}
/>
{/* 연속 번호 생성기 */}
<Drawer.Screen
name={Paths.LOTTO_MULTI}
component={MultiDrawComponent}
options={({ navigation }) => ({
title: "연속 행운번호 생성기",
drawerLabel: "연속 행운번호 생성기",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_MULTI}
/>
),
})}
/>
<Drawer.Screen
name={Paths.LOTTO_SAVED_NUMBERS}
component={SaveNumberHisScreen}
options={({ navigation }) => ({
title: "나의 행운번호 리포트",
drawerLabel: "나의 행운번호 리포트",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_SAVED_NUMBERS}
/>
),
})}
/>
<Drawer.Screen
name={Paths.LOTTO_NOTI}
component={LottoNotificationScreen}
options={({ navigation }) => ({
title: "알람 설정",
drawerLabel: "알람 설정",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_NOTI}
/>
),
})}
/>
</Drawer.Navigator>
);
};
export default DrawerNavigator;
5. 출력 결과 화면
5.1. 메인 화면에 Drawer 아이콘 생성
💡 메인 화면에 Drawer 아이콘 생성
- 위에 과정을 구성하게 되면 왼쪽에 Drawer 아이콘이 생깁니다.
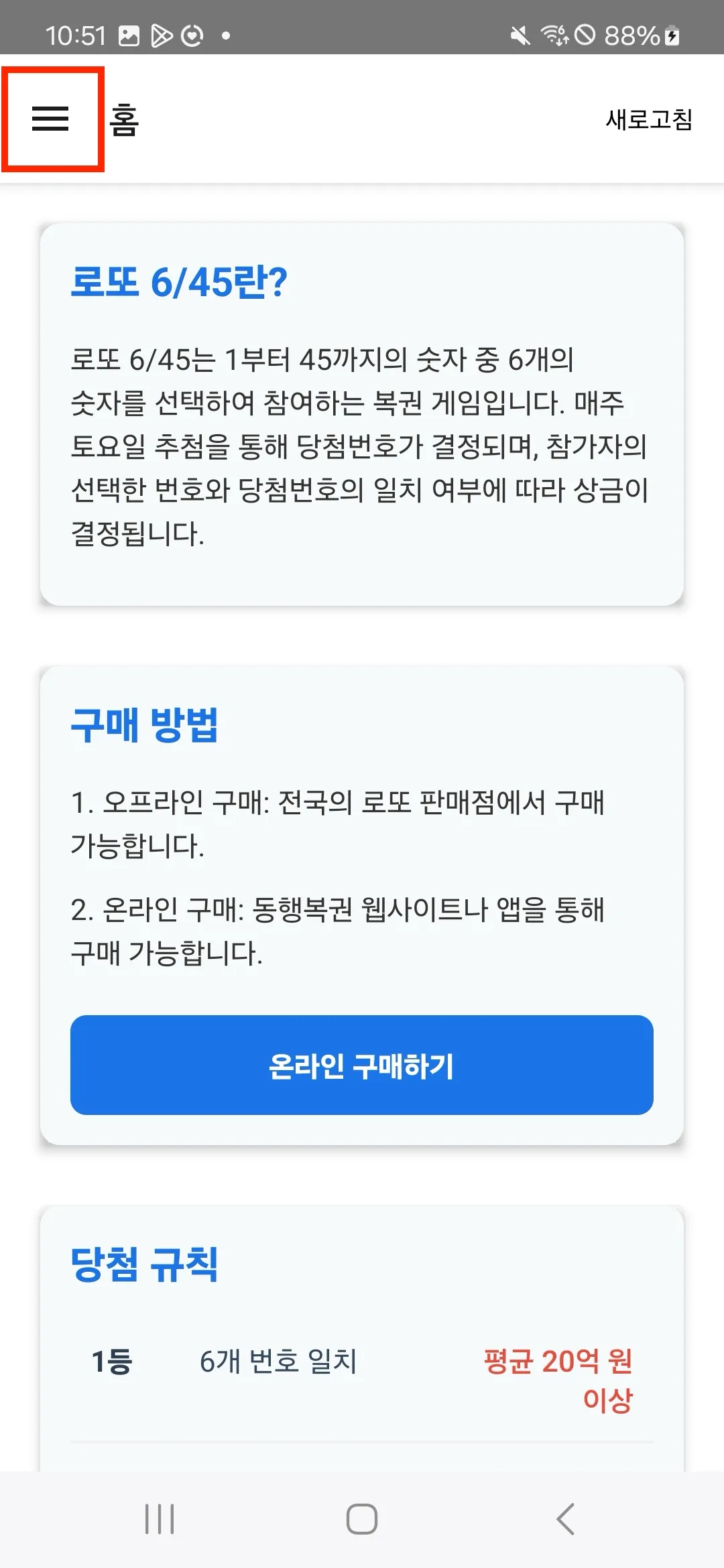
5.2. 아래와 같이 메뉴가 출력이 됨
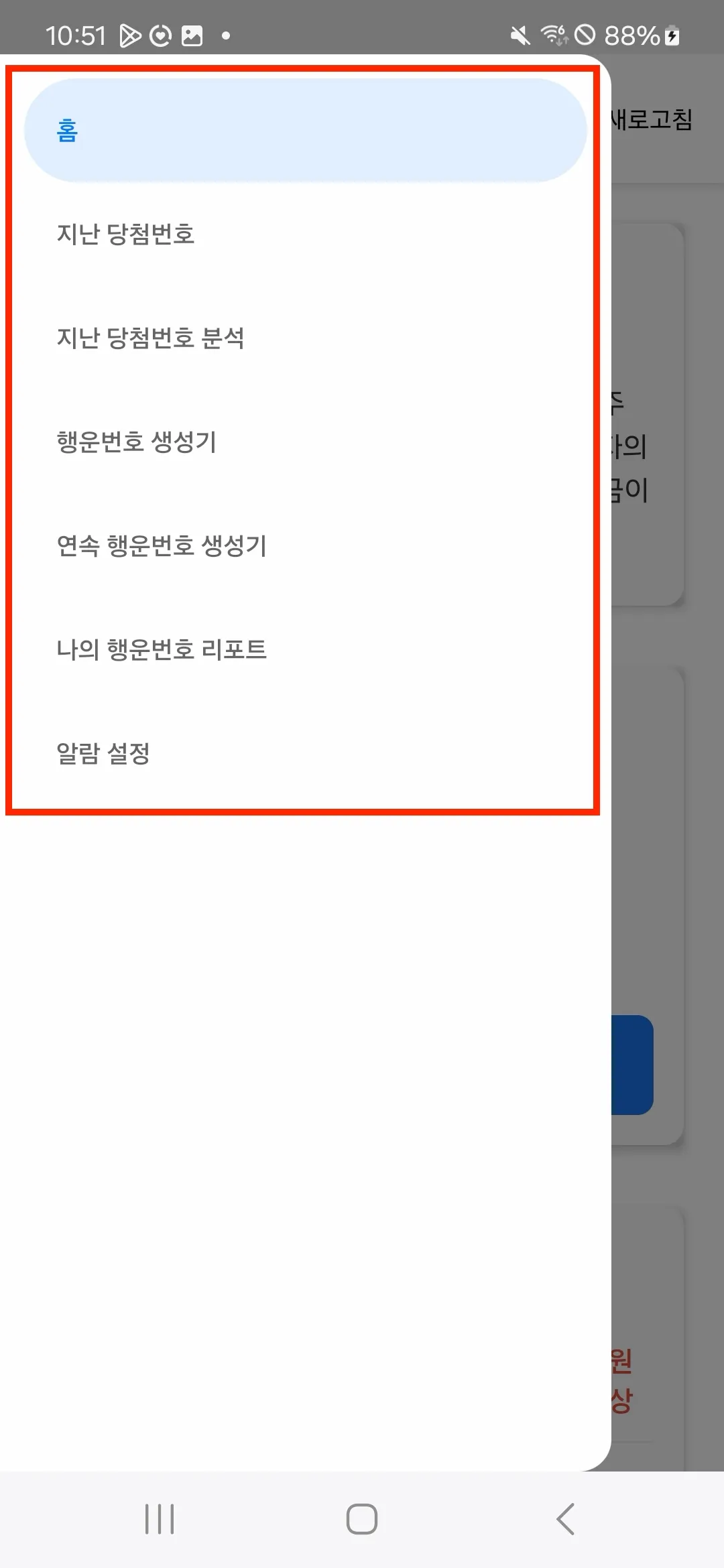
3) BottomTabNavigator와 함께 사용하기
1. DrawerNavigator
💡 DrawerNavigator
- 해당 부분은 Drawer Navigation과 Bottom Tab Navigation을 함께 사용하는 예시입니다. 즉, Drawer 메뉴가 출력이 되고 특정 페이지로 이동하였을 때, Bottom Tab Navigation가 출력이 되는 구조입니다.
- FortuneTabNavigator() 이라는 함수가 추가되었고, 해당 부분 내에 Bottom Tab Navigation을 사용하여 운세 관련 스크린들(음양오행, 십이지신, MBTI, 꿈, 별자리)을 하위 탭으로 구성하였습니다.
- DrawerNavigator 내에서는 component로 FortuneTabNavigator를 불러와서 출력을 합니다.
import ElementalLottoScreen from "@/screens/subfunc/ElementalLottoScreen";
import Home from "@/screens/Home";
import WinningHistoryScreens from "@/screens/WinningHistoryScreens";
import ZodiacLottoScreen from "@/screens/subfunc/ZodiacLottoScreen";
import { createDrawerNavigator } from "@react-navigation/drawer";
import { createBottomTabNavigator } from "@react-navigation/bottom-tabs";
import { Paths } from "./conf/Paths";
import DrawerContentScreen from "./screens/DrawerContentScreen";
import { Text, TouchableOpacity } from "react-native";
import MBTILottoScreen from "@/screens/subfunc/MbtiLottoScreen";
import DreamLottoScreen from "@/screens/subfunc/DreamLottoScreen";
import ConstellationLottoScreen from "@/screens/subfunc/ConstellationLottoScreen";
import MostWinningNumbersScreen from "@/screens/MostWinningNumbersScreen";
import SaveNumberHisScreen from "@/screens/SaveNumberHisScreen";
import NormalLottoScreen from "@/screens/NormalLottoScreen";
import MultiDrawComponent from "@/screens/MultiDrawComponent";
import LottoNotificationScreen from "@/screens/NotificationLottoScreen";
const Drawer = createDrawerNavigator();
const Tab = createBottomTabNavigator();
/**
* Drawer Navigator
* @returns
*/
const DrawerNavigator = () => {
// 새로고침 버튼 컴포넌트
const RefreshButton = ({ navigation, routeName }) => {
return (
<TouchableOpacity
onPress={() => {
navigation.reset({
index: 0,
routes: [{ name: routeName }],
});
}}
style={{ marginRight: 15 }}
>
<Text>새로고침</Text>
</TouchableOpacity>
);
};
// 운세 관련 탭 네비게이터 생성
const FortuneTabNavigator = () => {
return (
<Tab.Navigator>
<Tab.Screen
name="ElementalTab"
component={ElementalLottoScreen}
options={{ title: "음양오행" }}
/>
<Tab.Screen
name="ZodiacTab"
component={ZodiacLottoScreen}
options={{ title: "십이지신" }}
/>
<Tab.Screen
name="MBTITab"
component={MBTILottoScreen}
options={{ title: "MBTI" }}
/>
<Tab.Screen
name="DreamTab"
component={DreamLottoScreen}
options={{ title: "꿈" }}
/>
<Tab.Screen
name="ConstellationTab"
component={ConstellationLottoScreen}
options={{ title: "별자리" }}
/>
</Tab.Navigator>
);
};
return (
<Drawer.Navigator
initialRouteName={Paths.HOME}
backBehavior="history"
drawerContent={(props) => <DrawerContentScreen {...props} />}
screenOptions={{
drawerPosition: "left"
}}
>
{/* 메인 화면 */}
<Drawer.Screen
name={Paths.HOME}
component={Home}
options={({ navigation }) => ({
title: "홈",
drawerLabel: "홈",
headerRight: () => (
<RefreshButton navigation={navigation} routeName={Paths.HOME} />
),
})}
/>
{/* 당첨 번호 확인 */}
<Drawer.Screen
name={Paths.LOTTO_WINNING_HIS}
component={WinningHistoryScreens}
options={({ navigation }) => ({
title: "지난 당첨번호",
drawerLabel: "지난 당첨번호",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_WINNING_HIS}
/>
),
})}
/>
<Drawer.Screen
name={Paths.MOST_WINNING_HIS}
component={MostWinningNumbersScreen}
options={({ navigation }) => ({
title: "지난 당첨번호 분석",
drawerLabel: "지난 당첨번호 분석",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.MOST_WINNING_HIS}
/>
),
})}
/>
{/* 기본 번호 생성기 */}
<Drawer.Screen
name={Paths.LOTTO_NORMAL}
component={NormalLottoScreen}
options={({ navigation }) => ({
title: "행운번호 생성기",
drawerLabel: "행운번호 생성기",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_NORMAL}
/>
),
})}
/>
{/* 연속 번호 생성기 */}
<Drawer.Screen
name={Paths.LOTTO_MULTI}
component={MultiDrawComponent}
options={({ navigation }) => ({
title: "연속 행운번호 생성기",
drawerLabel: "연속 행운번호 생성기",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_MULTI}
/>
),
})}
/>
{/* 운세 번호 생성 */}
<Drawer.Screen
name={Paths.FORTUNE_NUMBERS}
component={FortuneTabNavigator}
options={({ navigation }) => ({
title: "나만의 맞춤 행운번호 생성기",
drawerLabel: "나만의 맞춤 행운번호 생성기",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.FORTUNE_NUMBERS}
/>
),
})}
/>
<Drawer.Screen
name={Paths.LOTTO_SAVED_NUMBERS}
component={SaveNumberHisScreen}
options={({ navigation }) => ({
title: "나의 행운번호 리포트",
drawerLabel: "나의 행운번호 리포트",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_SAVED_NUMBERS}
/>
),
})}
/>
<Drawer.Screen
name={Paths.LOTTO_NOTI}
component={LottoNotificationScreen}
options={({ navigation }) => ({
title: "알람 설정",
drawerLabel: "알람 설정",
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_NOTI}
/>
),
})}
/>
</Drawer.Navigator>
);
};
export default DrawerNavigator;
2. 결과 화면
2.1. 메인 화면에 Drawer 아이콘 생성
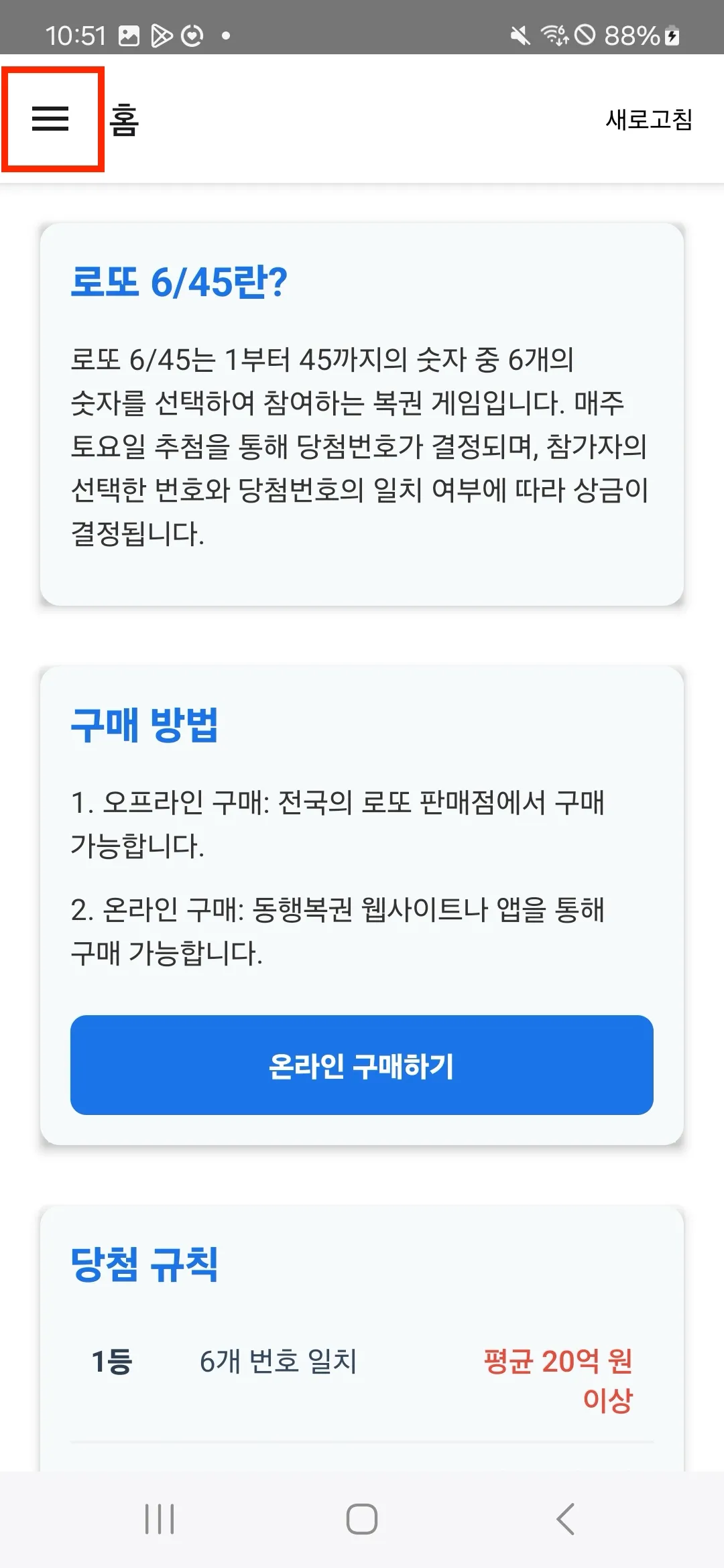
2.2. Drawer Navigation 내에 ‘나만의 맞춤 행운번호 생성기’ 탭을 추가하였습니다
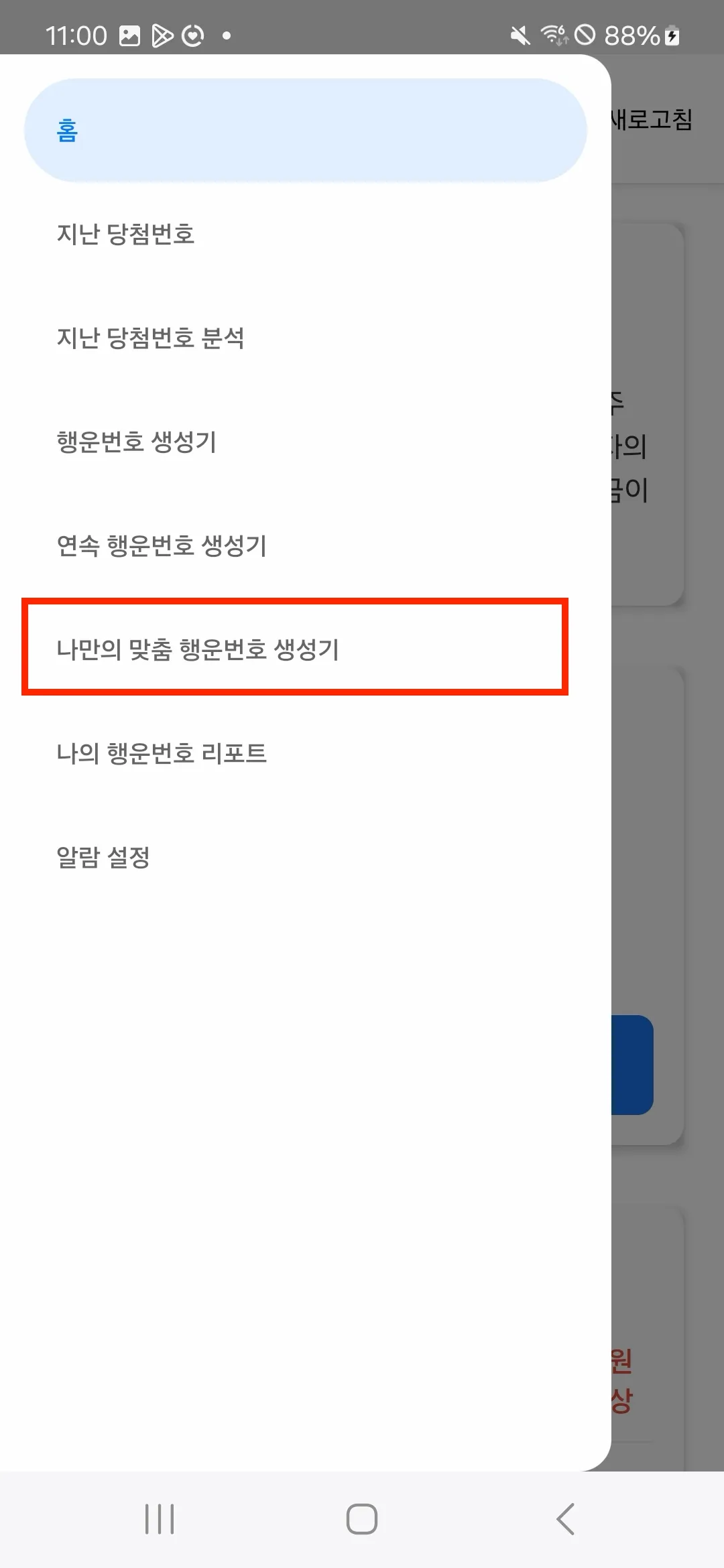
2.3. 아래와 같이 Bottom Tab Navigation이 출력이 됨을 확인하였습니다.
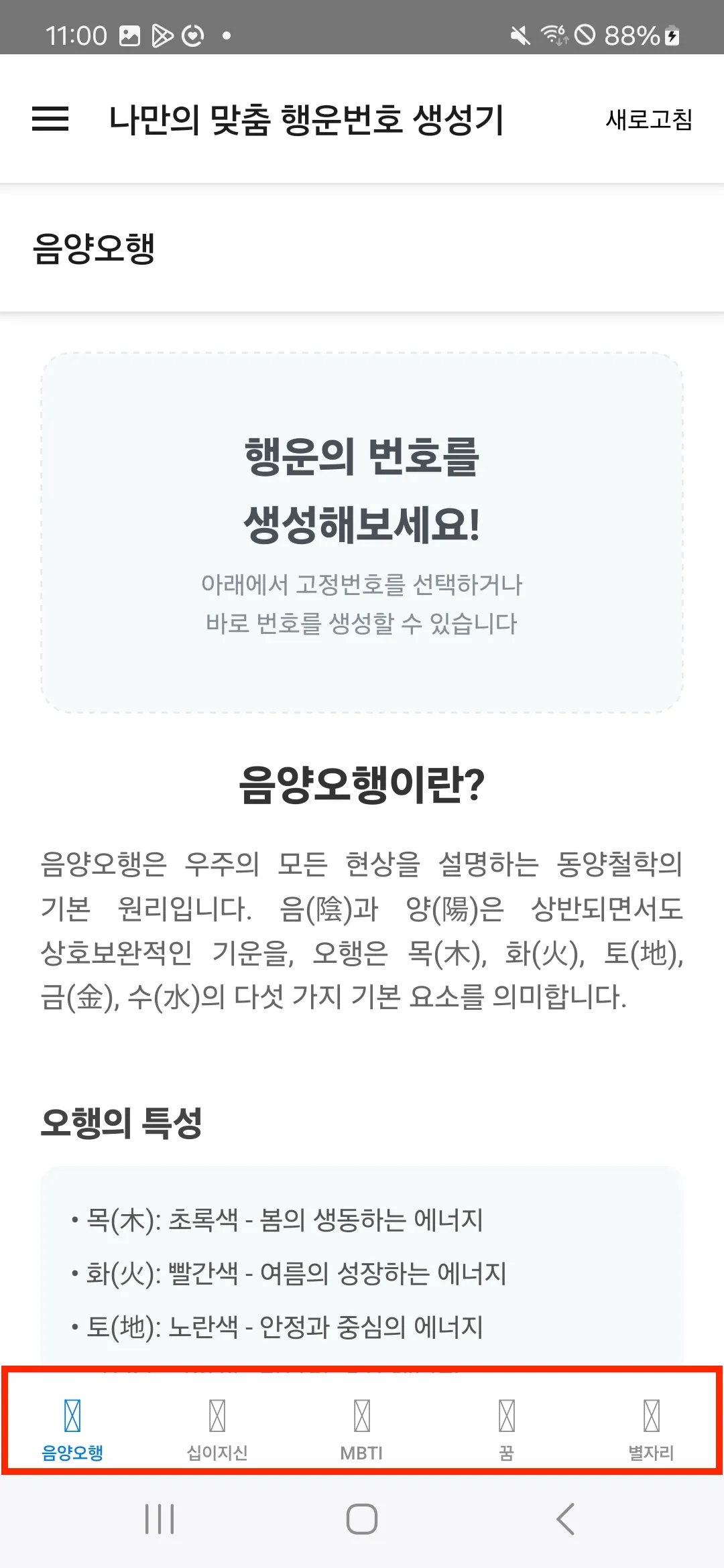
4) DrawerNavigator 내 아이콘과 함께 출력 : react-native-vector-icons 활용
💡 DrawerNavigator 내 아이콘과 함께 출력 : react-native-vector-icons 활용
- React Native 앱에서 사용할 수 있는 다양한 아이콘을 제공하는 인기 있는 라이브러리입니다.
특징 | 설명 |
다양한 아이콘 세트 | MaterialIcons, FontAwesome, Ionicons 등 여러 유명 아이콘 세트를 포함 |
커스터마이징 | 아이콘의 크기, 색상, 스타일을 쉽게 변경 가능 |
크로스 플랫폼 지원 | iOS와 Android 모두에서 동일하게 작동 |
최적화된 성능 | 벡터 기반 아이콘으로 다양한 해상도에서 선명하게 표시 |
💡 아래의 페이지 내에서 name 값을 찾아서 활용할 수 있습니다.
import Icon from 'react-native-vector-icons/MaterialIcons';
<Icon name="calendar-today" size={20} color="#fff" />
react-native-vector-icons directory
oblador.github.io
1. 라이브러리 추가
$ npm install react-native-vector-icons
# or
yarn add react-native-vector-icons
react-native-vector-icons
Customizable Icons for React Native with support for NavBar/TabBar, image source and full styling.. Latest version: 10.2.0, last published: 6 months ago. Start using react-native-vector-icons in your project by running `npm i react-native-vector-icons`. Th
www.npmjs.com
2. 추가 설정
2.1. Info.plist
<key>UIAppFonts</key>
<array>
<string>AntDesign.ttf</string>
<string>Entypo.ttf</string>
<string>EvilIcons.ttf</string>
<string>Feather.ttf</string>
<string>FontAwesome.ttf</string>
<string>FontAwesome5_Brands.ttf</string>
<string>FontAwesome5_Regular.ttf</string>
<string>FontAwesome5_Solid.ttf</string>
<string>FontAwesome6_Brands.ttf</string>
<string>FontAwesome6_Regular.ttf</string>
<string>FontAwesome6_Solid.ttf</string>
<string>Foundation.ttf</string>
<string>Ionicons.ttf</string>
<string>MaterialIcons.ttf</string>
<string>MaterialCommunityIcons.ttf</string>
<string>SimpleLineIcons.ttf</string>
<string>Octicons.ttf</string>
<string>Zocial.ttf</string>
<string>Fontisto.ttf</string>
</array>
2.2. build.gradle
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
3. 화면 적용
💡 화면 적용
- 기존의 화면에서 Drawer.Screen 태그 내에 drawerIcon 속성 값으로 <Icon> 태그를 추가하여 넣었습니다.
import ElementalLottoScreen from "@/screens/subfunc/ElementalLottoScreen";
import Home from "@/screens/Home";
import WinningHistoryScreens from "@/screens/WinningHistoryScreens";
import ZodiacLottoScreen from "@/screens/subfunc/ZodiacLottoScreen";
import { createDrawerNavigator } from "@react-navigation/drawer";
import { createBottomTabNavigator } from "@react-navigation/bottom-tabs";
import { Paths } from "./conf/Paths";
import DrawerContentScreen from "./screens/DrawerContentScreen";
import { Text, TouchableOpacity } from "react-native";
import MBTILottoScreen from "@/screens/subfunc/MbtiLottoScreen";
import DreamLottoScreen from "@/screens/subfunc/DreamLottoScreen";
import ConstellationLottoScreen from "@/screens/subfunc/ConstellationLottoScreen";
import MostWinningNumbersScreen from "@/screens/MostWinningNumbersScreen";
import SaveNumberHisScreen from "@/screens/SaveNumberHisScreen";
import NormalLottoScreen from "@/screens/NormalLottoScreen";
import MultiDrawComponent from "@/screens/MultiDrawComponent";
import LottoNotificationScreen from "@/screens/NotificationLottoScreen";
import Icon from 'react-native-vector-icons/MaterialIcons';
import { useFocusEffect } from '@react-navigation/native';
import { useCallback } from 'react';
const Drawer = createDrawerNavigator();
const Tab = createBottomTabNavigator();
/**
* Drawer Navigator
* @returns
*/
const DrawerNavigator = () => {
// 새로고침 버튼 컴포넌트
const RefreshButton = ({ navigation, routeName }) => {
return (
<TouchableOpacity
onPress={() => {
navigation.reset({
index: 0,
routes: [{ name: routeName }],
});
}}
style={{ marginRight: 15 }}
>
<Icon name="refresh" size={24} color="#000" />
</TouchableOpacity>
);
};
// 페이지 포커스 시 초기화하는 HOC
const withScreenReset = (WrappedComponent) => {
return (props) => {
useFocusEffect(
useCallback(() => {
// 화면이 포커스될 때 실행될 초기화 로직
props.navigation.setParams({
timestamp: Date.now() // 강제로 컴포넌트 리렌더링
});
return () => {
// cleanup 함수 (필요한 경우)
};
}, [])
);
return <WrappedComponent {...props} />;
};
};
// 운세 관련 탭 네비게이터 생성
const FortuneTabNavigator = () => {
return (
<Tab.Navigator>
<Tab.Screen
name="ElementalTab"
component={withScreenReset(ElementalLottoScreen)}
options={{
title: "음양오행",
tabBarIcon: ({ focused, color, size }) => (
<Icon name="balance" size={size} color={color} />
),
}}
/>
<Tab.Screen
name="ZodiacTab"
component={withScreenReset(ZodiacLottoScreen)}
options={{
title: "십이지신",
tabBarIcon: ({ focused, color, size }) => (
<Icon name="pets" size={size} color={color} />
),
}}
/>
<Tab.Screen
name="MBTITab"
component={withScreenReset(MBTILottoScreen)}
options={{
title: "MBTI",
tabBarIcon: ({ focused, color, size }) => (
<Icon name="psychology" size={size} color={color} />
),
}}
/>
<Tab.Screen
name="DreamTab"
component={withScreenReset(DreamLottoScreen)}
options={{
title: "꿈",
tabBarIcon: ({ focused, color, size }) => (
<Icon name="cloud" size={size} color={color} />
),
}}
/>
<Tab.Screen
name="ConstellationTab"
component={withScreenReset(ConstellationLottoScreen)}
options={{
title: "별자리",
tabBarIcon: ({ focused, color, size }) => (
<Icon name="star" size={size} color={color} />
),
}}
/>
</Tab.Navigator>
);
};
return (
<Drawer.Navigator
initialRouteName={Paths.HOME}
backBehavior="history"
drawerContent={(props) => <DrawerContentScreen {...props} />}
screenOptions={{
drawerPosition: "left"
}}
>
{/* 메인 화면 */}
<Drawer.Screen
name={Paths.HOME}
component={withScreenReset(Home)}
options={({ navigation }) => ({
title: "홈",
drawerLabel: "홈",
drawerIcon: ({ color, size }) => (
<Icon name="home" size={size} color={color} />
),
headerRight: () => (
<RefreshButton navigation={navigation} routeName={Paths.HOME} />
),
})}
/>
{/* 당첨 번호 확인 */}
<Drawer.Screen
name={Paths.LOTTO_WINNING_HIS}
component={withScreenReset(WinningHistoryScreens)}
options={({ navigation }) => ({
title: "지난 당첨번호",
drawerLabel: "지난 당첨번호",
drawerIcon: ({ color, size }) => (
<Icon name="history" size={size} color={color} />
),
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_WINNING_HIS}
/>
),
})}
/>
<Drawer.Screen
name={Paths.MOST_WINNING_HIS}
component={withScreenReset(MostWinningNumbersScreen)}
options={({ navigation }) => ({
title: "지난 당첨번호 분석",
drawerLabel: "지난 당첨번호 분석",
drawerIcon: ({ color, size }) => (
<Icon name="analytics" size={size} color={color} />
),
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.MOST_WINNING_HIS}
/>
),
})}
/>
{/* 기본 번호 생성기 */}
<Drawer.Screen
name={Paths.LOTTO_NORMAL}
component={withScreenReset(NormalLottoScreen)}
options={({ navigation }) => ({
title: "행운번호 생성기",
drawerLabel: "행운번호 생성기",
drawerIcon: ({ color, size }) => (
<Icon name="casino" size={size} color={color} />
),
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_NORMAL}
/>
),
})}
/>
{/* 연속 번호 생성기 */}
<Drawer.Screen
name={Paths.LOTTO_MULTI}
component={withScreenReset(MultiDrawComponent)}
options={({ navigation }) => ({
title: "연속 행운번호 생성기",
drawerLabel: "연속 행운번호 생성기",
drawerIcon: ({ color, size }) => (
<Icon name="format-list-numbered" size={size} color={color} />
),
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_MULTI}
/>
),
})}
/>
{/* 운세 번호 생성 */}
<Drawer.Screen
name={Paths.FORTUNE_NUMBERS}
component={FortuneTabNavigator}
options={({ navigation }) => ({
title: "나만의 맞춤 행운번호 생성기",
drawerLabel: "나만의 맞춤 행운번호 생성기",
drawerIcon: ({ color, size }) => (
<Icon name="auto-awesome" size={size} color={color} />
),
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.FORTUNE_NUMBERS}
/>
),
})}
/>
<Drawer.Screen
name={Paths.LOTTO_SAVED_NUMBERS}
component={withScreenReset(SaveNumberHisScreen)}
options={({ navigation }) => ({
title: "나의 행운번호 리포트",
drawerLabel: "나의 행운번호 리포트",
drawerIcon: ({ color, size }) => (
<Icon name="assessment" size={size} color={color} />
),
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_SAVED_NUMBERS}
/>
),
})}
/>
<Drawer.Screen
name={Paths.LOTTO_NOTI}
component={withScreenReset(LottoNotificationScreen)}
options={({ navigation }) => ({
title: "알람 설정",
drawerLabel: "알람 설정",
drawerIcon: ({ color, size }) => (
<Icon name="notifications" size={size} color={color} />
),
headerRight: () => (
<RefreshButton
navigation={navigation}
routeName={Paths.LOTTO_NOTI}
/>
),
})}
/>
</Drawer.Navigator>
);
};
export default DrawerNavigator;
4. 결과 화면
💡 결과 화면
- 아래와 같이 Drawer 메뉴 내에 아이콘이 함께 출력됨을 확인하였습니다.
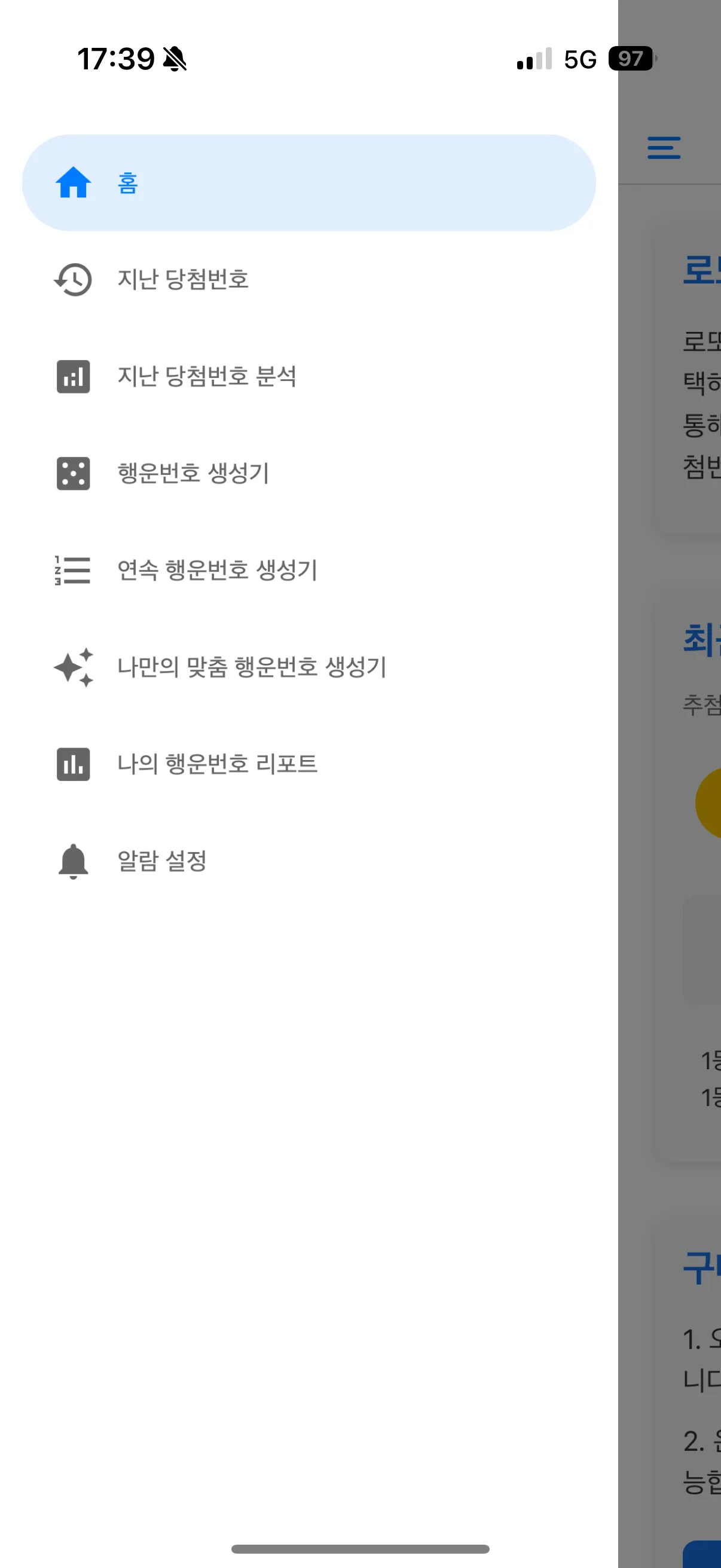
오늘도 감사합니다. 😀